Golang中的加密與解密實踐
Golang中的加密與解密實踐
隨著互聯網的不斷發展,越來越多的信息需要傳輸,而這些信息中很多都是敏感信息,為了保證信息安全,加密技術就被廣泛應用。在Golang中,我們可以通過各種加密算法來保證信息安全,同時也可以通過相應的解密算法來還原加密后的信息。本文將介紹一些常見的加密與解密算法,并提供相應的代碼示例。
一、MD5加密算法
MD5是一種哈希算法,它將任意長度的二進制值映射為一個128位的小寫字母和數字組成的字符串。MD5算法具有抗碰撞特性,即不同的輸入得到的哈希值很難相同。在Golang中,我們可以通過crypto/md5包來實現MD5加密算法。
示例代碼:
`go
import (
"crypto/md5"
"encoding/hex"
"fmt"
)
// 對字符串進行MD5加密
func Md5Encrypt(str string) string {
md5Ctx := md5.New()
md5Ctx.Write(byte(str))
cipherStr := md5Ctx.Sum(nil)
return hex.EncodeToString(cipherStr)
}
func main() {
str := "hello world"
encryptedStr := Md5Encrypt(str)
fmt.Println("加密前:", str)
fmt.Println("加密后:", encryptedStr)
}
二、AES加密算法AES是一種對稱加密算法,它是目前最流行的加密算法之一,具有高效、安全、可靠等特點。在Golang中,我們可以通過crypto/aes包來實現AES加密算法。示例代碼:`goimport ( "bytes" "crypto/aes" "crypto/cipher" "encoding/base64" "fmt") // 對字符串進行AES加密func AesEncrypt(str, key string) (string, error) { strBytes := byte(str) keyBytes := byte(key) block, err := aes.NewCipher(keyBytes) if err != nil { return "", err } blockSize := block.BlockSize() strBytes = PKCS5Padding(strBytes, blockSize) iv := keyBytes blockMode := cipher.NewCBCEncrypter(block, iv) cipherText := make(byte, len(strBytes)) blockMode.CryptBlocks(cipherText, strBytes) return base64.StdEncoding.EncodeToString(cipherText), nil} // 對字符串進行AES解密func AesDecrypt(str, key string) (string, error) { strBytes, err := base64.StdEncoding.DecodeString(str) if err != nil { return "", err } keyBytes := byte(key) block, err := aes.NewCipher(keyBytes) if err != nil { return "", err } blockSize := block.BlockSize() iv := keyBytes blockMode := cipher.NewCBCDecrypter(block, iv) origData := make(byte, len(strBytes)) blockMode.CryptBlocks(origData, strBytes) origData = PKCS5UnPadding(origData) return string(origData), nil} // PKCS5填充func PKCS5Padding(cipherText byte, blockSize int) byte { padding := blockSize - len(cipherText)%blockSize padText := bytes.Repeat(byte{byte(padding)}, padding) return append(cipherText, padText...)} // PKCS5去除填充func PKCS5UnPadding(origData byte) byte { length := len(origData) unPadding := int(origData) return origData} func main() { str := "hello world" key := "1234567890123456" encryptedStr, err := AesEncrypt(str, key) if err != nil { fmt.Println("加密失敗:", err) return } decryptedStr, err := AesDecrypt(encryptedStr, key) if err != nil { fmt.Println("解密失敗:", err) return } fmt.Println("加密前:", str) fmt.Println("加密后:", encryptedStr) fmt.Println("解密后:", decryptedStr)}
三、RSA加密算法
RSA是一種非對稱加密算法,它通過公鑰加密、私鑰解密的方式實現信息的安全傳輸。在Golang中,我們可以通過crypto/rsa包來實現RSA加密算法。
示例代碼:
`go
import (
"crypto/rand"
"crypto/rsa"
"crypto/x509"
"encoding/base64"
"encoding/pem"
"fmt"
)
// 生成RSA密鑰對
func GenerateRsaKeyPair() (*rsa.PrivateKey, error) {
privateKey, err := rsa.GenerateKey(rand.Reader, 2048)
if err != nil {
return nil, err
}
return privateKey, nil
}
// 使用公鑰進行RSA加密
func RsaEncrypt(plainText byte, publicKey byte) (byte, error) {
block, _ := pem.Decode(publicKey)
if block == nil {
return nil, fmt.Errorf("public key error")
}
pubInterface, err := x509.ParsePKIXPublicKey(block.Bytes)
if err != nil {
return nil, err
}
pub := pubInterface.(*rsa.PublicKey)
cipherText, err := rsa.EncryptPKCS1v15(rand.Reader, pub, plainText)
if err != nil {
return nil, err
}
return cipherText, nil
}
// 使用私鑰進行RSA解密
func RsaDecrypt(cipherText byte, privateKey byte) (byte, error) {
block, _ := pem.Decode(privateKey)
if block == nil {
return nil, fmt.Errorf("private key error")
}
priv, err := x509.ParsePKCS1PrivateKey(block.Bytes)
if err != nil {
return nil, err
}
plainText, err := rsa.DecryptPKCS1v15(rand.Reader, priv, cipherText)
if err != nil {
return nil, err
}
return plainText, nil
}
func main() {
str := "hello world"
privateKey, err := GenerateRsaKeyPair()
if err != nil {
fmt.Println("生成密鑰對失敗:", err)
return
}
publicKey := &privateKey.PublicKey
cipherText, err := RsaEncrypt(byte(str), byte(publicKey))
if err != nil {
fmt.Println("加密失敗:", err)
return
}
plainText, err := RsaDecrypt(cipherText, byte(privateKey))
if err != nil {
fmt.Println("解密失敗:", err)
return
}
fmt.Println("加密前:", str)
fmt.Println("加密后:", base64.StdEncoding.EncodeToString(cipherText))
fmt.Println("解密后:", string(plainText))
}
四、總結
本文介紹了Golang中常見的加密與解密算法,包括MD5、AES、RSA等。這些加密算法在實際開發中非常常見,開發者需要在保證信息安全的前提下,選擇合適的加密算法來保護數據的安全。同時,本文提供了相應的代碼示例,讀者可以根據需要進行調整和使用。

相關推薦HOT
更多>>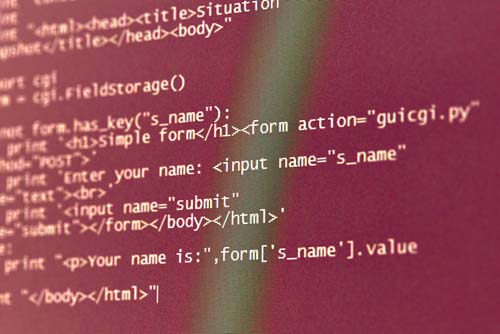
Golang高速并發編程(二)
Golang高速并發編程(二)在上一篇文章中,我們已經初步探討了Golang在高速并發編程方面的優勢以及如何通過Golang實現高并發。接下來,我們將進...詳情>>
2023-12-27 23:51:18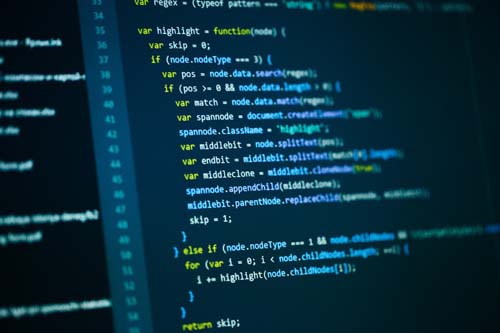
Golang中的函數式編程實踐
Golang 中的函數式編程實踐在現代編程語言中,函數式編程已經成為了一種非常流行的編程范式。它被廣泛應用于各種應用程序的開發中,尤其在數據...詳情>>
2023-12-27 15:27:17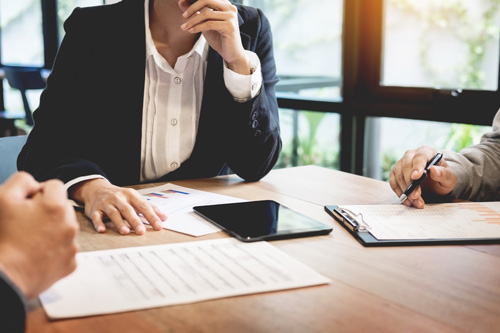
如何避免惡意軟件和病毒攻擊?
如何避免惡意軟件和病毒攻擊?惡意軟件和病毒攻擊是互聯網時代最為常見的威脅之一。一旦計算機受到攻擊,便有可能導致數據泄露或計算機系統完全...詳情>>
2023-12-27 04:39:17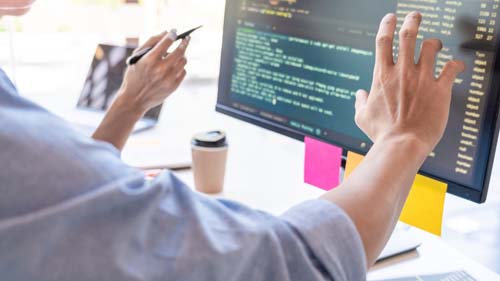
服務器安全漏洞排查方法大全!
服務器安全漏洞排查方法大全!在今天的互聯網時代,服務器安全是一項十分重要的任務。一旦服務器出現漏洞,就會對網站造成不可預估的損失,甚至...詳情>>
2023-12-26 20:15:16